Accessing the GraphQL endpoint
In the following sections we look specifically at how to access the GraphQL endpoint with calls from external systems. First we look at accessing the endpoint via EDGs Swagger API and cURL. Next, how to call the endpoint with some sample Python code (this will require a basic understanding of Python). To find out more about using GraphQL and Topbraids GraphiQL client, see GraphQL Queries documentation.
To learn more about GraphQL in EDG, please first consult the GraphQL documentation.
Contents
1. Accessing GraphQL via Swagger and cURL
In this section you will learn to navigate to Swaggers GraphQL endpoint and query over EDG’s sample data. We will be looking specifically at the “Kennedy Family” Data Graph.
To learn more about accessing and using Swagger in EDG, please consult the following documentation: Web services and Swagger and Swagger References.
The first step is to access the reports tab in your target asset collection, i.e. the asset collection which you wish to run your GraphQL query. So, first select the Kennedy Family data graph, go to the reports tab and select “Web Services Swagger UI”, and navigate to “GraphQL”.

The GraphQL Swagger endpoint in the reports tab for the Kennedy Family asset collection
Under GraphQL you will see three options. These three options are related to the different available schemas, i.e. tbl/graphql/_
is for making queries against the edg platform, tbl/graphql/kennedy_family
for making queries over the kennedy_family asset collection, and tbl/graphql/kennedy_family/{schema}
is for making queries against specific schemas (i.e. any class or shape in your asset collection). You can find out more about these in the GraphQL Queries documentation.
Here we will look at making a basic query over the graphql/kennedy_family endpoint, so click on that option and then click “Try it out”.
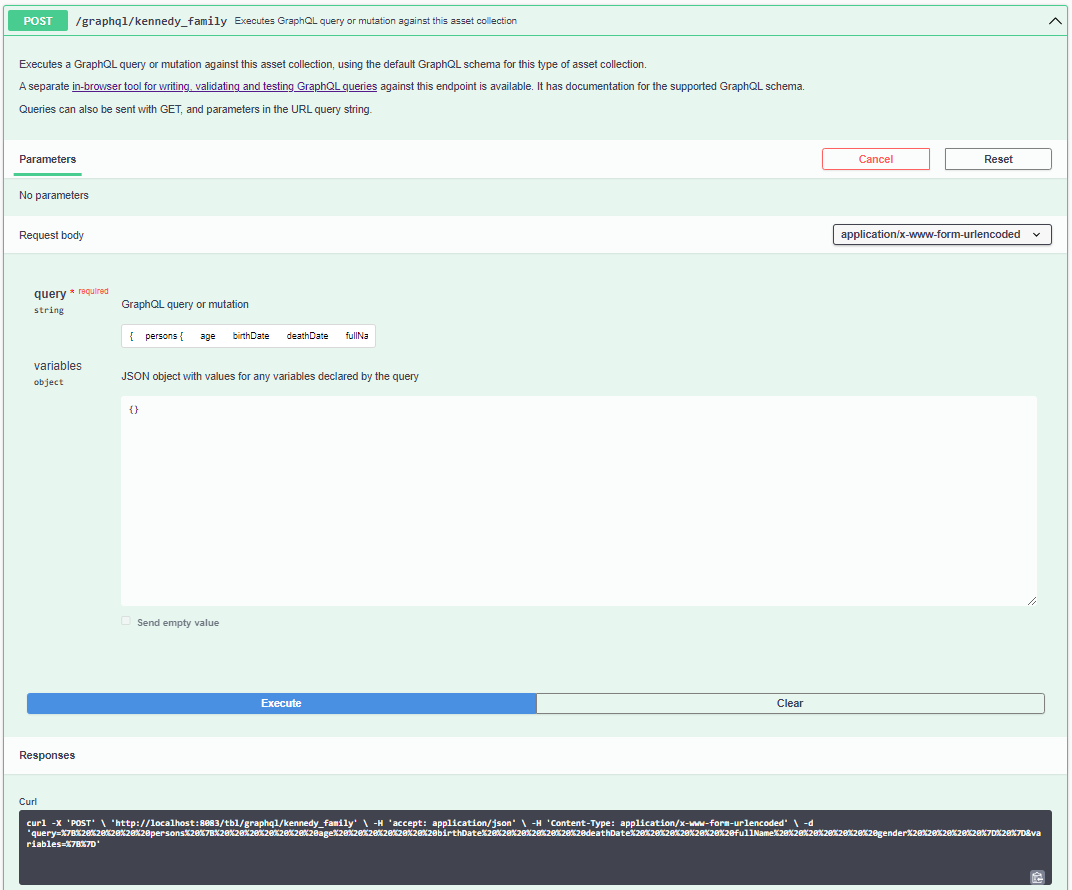
An example of a simple GraphQL query over the Kennedy Family asset collection
Then in the “query” field add the below GraphQL query. This query returns all instances of “Person” along with any available values for birthDate, deathDate, fullName and gender. You can remove spaces to avoid the use of escape characters in the resulting cURL (see below).
{
persons {
birthDate
deathDate
fullName
gender
}
}
Once you execute you will see a response as a list of JSON values:
{
"data": {
"persons": [
{
"birthDate": "1947-07-30",
"deathDate": null,
"fullName": "Arnold Schwarzenegger",
"gender": "male"
},
{
"birthDate": null,
"deathDate": null,
"fullName": "Maria Shriver",
"gender": "female"
},
...
You will also notice that Swagger provides an example cURL command for the input GraphQL query (you will need to edit this accordingly to run via windows command).
curl -X 'POST' \
'http://localhost:8083/tbl/graphql/kennedy_family' \
-H 'accept: application/json' \
-H 'Content-Type: application/x-www-form-urlencoded' \
-d 'query=%7Bpersons%20%7BbirthDate%20deathDate%20fullName%20gender%7D%20%7D&variables=%7B%7D'
When running EDG on SaaS you would replace “localhost:8083” with the URL of your server. This also assumes you are running on localhost with no authentication. All the following examples assume no authentication, but in the next section, we shall describe how to account for different types of authentication in EDG. For more on the differents methods for authenticating, please see the documentation on authentication
1.1 Using authentication in EDG
In this section we cover making calls to the GraphQL endpoint using three different forms of authentication. We also provide reference to Python scripts for each form of authentication, which can be found here Accessing EDG APIs with different types of authentication
Basic and SAML based authentication
Form-based Authentication
0Auth Authentication
1.1.1 Basic and SAML based authentication
Basic authentication relies on a Base64 encoded Authorization
header whose value consists of the word Basic
followed by a space followed by the Base64 encoded name:password
. This is better suited for service-only access because the only way a user can log out is to shut down their browser. The URL with header information can be submitted with authentication information. Here’s an example using cURL to access using the previous example to query the GraphQL endpoint.
curl -X 'POST' \
'http://localhost:8083/tbl/graphql/kennedy_family' \
-H 'accept: application/json' \
-H 'Authorization: Basic c2NvdHQ6MTIzNDU=' \
-H 'Content-Type: application/x-www-form-urlencoded' \
-d 'query=%7Bpersons%20%7BbirthDate%20deathDate%20fullName%20gender%7D%20%7D&variables=%7B%7D'
The same approach should also work for SAML based authentication. To try this out using Python, see Using basic and saml based authentication. For secure access, web services should use HTTPS encryption.
1.1.2 Form-based Authentication Script
Access using Form-based authentication, while not recommended, is possible using cookies generated by the server. One method is to respond to the challenge with a hardcoded URL with a valid user id and password. The general form of this response is:
http://[host]:[port]/edg/j_security_check?j_username=[username]&j_password=[password]
Another method is to request an HTTP cookie that can be used in subsequent requests. The following is an example script for accessing a TopBraid form-based server. The script interaction has three parts:
The client requests a cookie, e.g.,
loginRequestCookie
, to use for logging in usingGET
orPOST
. Replace $serverURL with your server URL, e.g. “http://localhost:8083/tbl”:
# Return the loginRequestCookie
curl --cookie-jar loginRequestCookie --request POST "$serverURL"
The client logs in, and if successful, the client will receive another cookie, e.g.,
loginSuccessCookie
, which is saved for subsequent requests. Replace username and password with your username and password, e.g. admin1 and password1. Use the same serverURL as above.
# Generate the loginSuccessCookie
curl --include --cookie loginRequestCookie \
--data-urlencode "j_username=$username" \
--data-urlencode "j_password=$password" \
--location "$serverURL/swp?_viewName=home" \
--location "$serverURL/j_security_check" \
--cookie-jar loginSuccessCookie
The client uses the
loginSuccessCookie
for subsequent TopBraid service requests, such as the GraphQL endpoint call in the example. Use the same serverURL as above. You can also provide variables for the graph name, as well as the query if you wish to pass this into a bash script.
# Use the loginSuccessCookie to query GraphQL
curl --cookie loginSuccessCookie \
--request POST "$serverURL/graphql/kennedy_family" \
--data-urlencode "query={persons{birthDate deathDate fullName gender}}" \
--data "format=json"
To try this out using Python, see Using Form-based authentication. For secure access, web services should use HTTPS encryption.
1.1.3 0Auth Authentication Script
When using 0Auth authentication, we recommend you make use of our python script available here - Using 0auth authentication
1. Accessing GraphQL using a Python Script
In this section we take the above GraphQL endpoint and write a piece of Python script to allow you to make a call to EDG, which takes as parameters the URL of your server, the asset collection, and the query. It assumes you are using no authentication, see the previous section for handling authentication. The python code will need to import two libraries, requests (to handle the HTTP requests) and sys (to handle input parameters). Next we write a main function, which first checks we have provided the correct number of parameters. If yes, it takes these, stores them as variables, and passes them to a function “graphql_query”.
import requests
import argparse
def main():
parser = argparse.ArgumentParser()
parser.add_argument("INPUT_URL", help="the edg instance host")
parser.add_argument("ASSET_COLLECTION", help="the asset collection to query")
parser.add_argument("QUERY", help="the query string")
args = parser.parse_args()
graphql_query(args.INPUT_URL, args.ASSET_COLLECTION, args.QUERY)
Now we write the graphql_query function. This function takes the input url, the name of the asset collection, and finally the GraphQL query and uses these to generate a HTTP post request. If successful, it returns the response json, and if not it generates an error message.
def graphql_query(input_url, asset_collection, query):
url = f"{input_url}/tbl/graphql/{asset_collection}"
headers = {
"accept": "application/json",
"Content-Type": "application/x-www-form-urlencoded",
}
payload = {"query": query, "variables": "{}"}
response = requests.post(url, headers=headers, data=payload)
if response.status_code == 200:
return response.json()
else:
error_message = (
f"Request failed with status code {response.status_code}: {response.text}"
)
raise Exception(error_message)
You can download the complete code example here graphql_query.py
To test this, running EDG Studio locally, use the following command -
python graphql_query.py "http://localhost:8083" "kennedy_family" "{persons {birthDate deathDate fullName gender} }"